일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | ||||
4 | 5 | 6 | 7 | 8 | 9 | 10 |
11 | 12 | 13 | 14 | 15 | 16 | 17 |
18 | 19 | 20 | 21 | 22 | 23 | 24 |
25 | 26 | 27 | 28 | 29 | 30 | 31 |
Tags
- Spring
- 이펙티브자바
- springboot
- mybatis
- Greedy
- SQL
- 알고리즘
- 프로그래머스
- java
- IntelliJ
- db
- BFS
- select
- 탐욕법
- mariaDB
- 깊이우선탐색
- Database
- DFS
- 우선순위큐
- 정렬
- 피보나치
- Effective Java
- DP
- join
- 다이나믹프로그래밍
- 백준
- 너비우선탐색
- 데이터베이스
- 코테
- 그리디알고리즘
Archives
- Today
- Total
땀두 블로그
[백준] 10026번 - 적록색약 본문
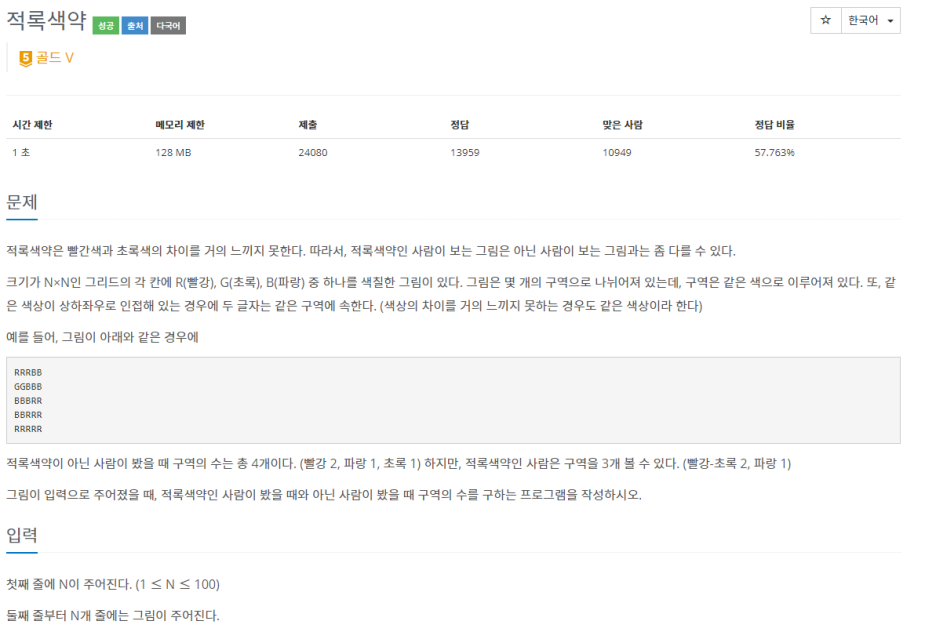
간단한 BFS문제이다. 처음 탐색 이후 해당 배열을 clone해주고, R이나 G를 하나의 값으로 합쳐준 다음 탐색을 해주면 된다.
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.LinkedList;
import java.util.Queue;
class Node10026 {
int x;
int y;
Node10026(int x, int y) {
this.x = x;
this.y = y;
}
}
public class p10026 {
public static boolean[][] visited;
public static int a;
public static int[] nx = { -1, 0, 0, 1 };
public static int[] ny = { 0, -1, 1, 0 };
public static int cnt = 0;
public static void main(String[] args) throws IOException {
// TODO Auto-generated method stub
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
a = Integer.parseInt(br.readLine());
String[][] s = new String[a][a];
for (int i = 0; i < a; i++) {
s[i] = br.readLine().split("");
}
visited = new boolean[a][a];
for (int i = 0; i < a; i++) {
for (int j = 0; j < a; j++) {
if (visited[i][j] == false) {
bfs(i, j, s);
}
}
}
System.out.print(cnt);
cnt = 0;
String[][] ary = s.clone();
for (int i = 0; i < a; i++) {
for (int j = 0; j < a; j++) {
if (ary[i][j].equals("R")) {
ary[i][j] = "G";
}
}
}
visited = new boolean[a][a];
for (int i = 0; i < a; i++) {
for (int j = 0; j < a; j++) {
if (visited[i][j] == false) {
bfs(i, j, ary);
}
}
}
System.out.println(" " + cnt);
}
public static void bfs(int x, int y, String[][] s) {
Queue<Node10026> q = new LinkedList<>();
q.add(new Node10026(x, y));
visited[x][y] = true;
while (!q.isEmpty()) {
Node10026 n = q.poll();
for (int i = 0; i < 4; i++) {
int X = n.x + nx[i];
int Y = n.y + ny[i];
if (X >= 0 && Y >= 0 && X < a && Y < a) {
if (s[x][y].equals(s[X][Y]) && visited[X][Y] == false) {
visited[X][Y] = true;
q.add(new Node10026(X, Y));
}
}
}
}
cnt++;
}
}
'알고리즘 > 백준' 카테고리의 다른 글
[백준] 16236번 - 아기상어 (0) | 2022.03.23 |
---|---|
[백준] 14500번 - 테트로미노 (0) | 2022.03.23 |
[백준] 9019번 - DSLR (0) | 2022.03.23 |
[백준] 5430번 - AC (0) | 2022.03.23 |
[백준] 1107번 - 리모콘 (0) | 2022.03.23 |
Comments